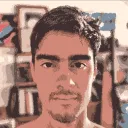
java
@Getter
public class Credentials {
private final String clientId;
private final String clientSecret;
private final String certificate;
private final boolean sandbox;
private final boolean debug;
public Credentials() {
ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
InputStream credentialsFile = classLoader.getResourceAsStream("credentials.json");
assert credentialsFile != null;
JSONTokener tokenizer = new JSONTokener(credentialsFile);
JSONObject credentials = new JSONObject(tokenizer);
try {
credentialsFile.close();
} catch (IOException e) {
System.out.println("Impossible to close file credentials.json");
}
this.clientId = credentials.getString("client_id");
this.clientSecret = credentials.getString("client_secret");
this.certificate = credentials.getString("certificate");
this.sandbox = credentials.getBoolean("sandbox");
this.debug = credentials.getBoolean("debug");
}
public HashMap getOptionsMap() {
HashMap options = new HashMap<>();
options.put("client_id", this.getClientId());
options.put("client_secret", this.getClientSecret());
options.put("certificate", this.getCertificate());
options.put("sandbox", this.isSandbox());
return options;
}
public JSONObject getOptionsJson() {
return new JSONObject(this.getOptionsMap());
}
}
@Getter
public class Credentials {
private final String clientId;
private final String clientSecret;
private final String certificate;
private final boolean sandbox;
private final boolean debug;
public Credentials() {
ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
InputStream credentialsFile = classLoader.getResourceAsStream("credentials.json");
assert credentialsFile != null;
JSONTokener tokenizer = new JSONTokener(credentialsFile);
JSONObject credentials = new JSONObject(tokenizer);
try {
credentialsFile.close();
} catch (IOException e) {
System.out.println("Impossible to close file credentials.json");
}
this.clientId = credentials.getString("client_id");
this.clientSecret = credentials.getString("client_secret");
this.certificate = credentials.getString("certificate");
this.sandbox = credentials.getBoolean("sandbox");
this.debug = credentials.getBoolean("debug");
}
public HashMap getOptionsMap() {
HashMap options = new HashMap<>();
options.put("client_id", this.getClientId());
options.put("client_secret", this.getClientSecret());
options.put("certificate", this.getCertificate());
options.put("sandbox", this.isSandbox());
return options;
}
public JSONObject getOptionsJson() {
return new JSONObject(this.getOptionsMap());
}
}